Sign in to follow this
Followers
0
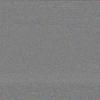
Need some help with c++ code
Started by
Hellu,
3 posts in this topic
Create an account or sign in to comment
You need to be a member in order to leave a comment
Sign in to follow this
Followers
0